Payment Service
Overview
The Payment Service offers a fully customizable payment flow that gives businesses complete control over the checkout process. Designed for companies that need a branded and flexible payment experience, this service allows you to build a native, seamless checkout directly within your app or website.
Customizable Payment Flow
With the Payment Service, merchants can create a branded checkout experience entirely within their environment. The payment process is integrated into the merchant's app or website, allowing for full customization of the user interface and flow.
Ideal for Full Control
This service is best suited for businesses that require a custom payment solution tailored to their unique needs. It allows for deep customization, making it ideal for large businesses or apps that need to manage every detail of the payment process.
Integration Flexibility
Unlike the Checkout Service, which uses a pre-built hosted page, the Payment Service allows developers to build and control the entire payment experience. This includes managing payment methods, user experience, and handling success or failure responses directly within the app.
Use Case
Ideal for large-scale applications, e-commerce platforms, or businesses that require a complex, branded checkout experience with full control over the payment journey.
Integration flow
The sequence diagram below illustrates the flow for integrating Payment service via the Web SDK
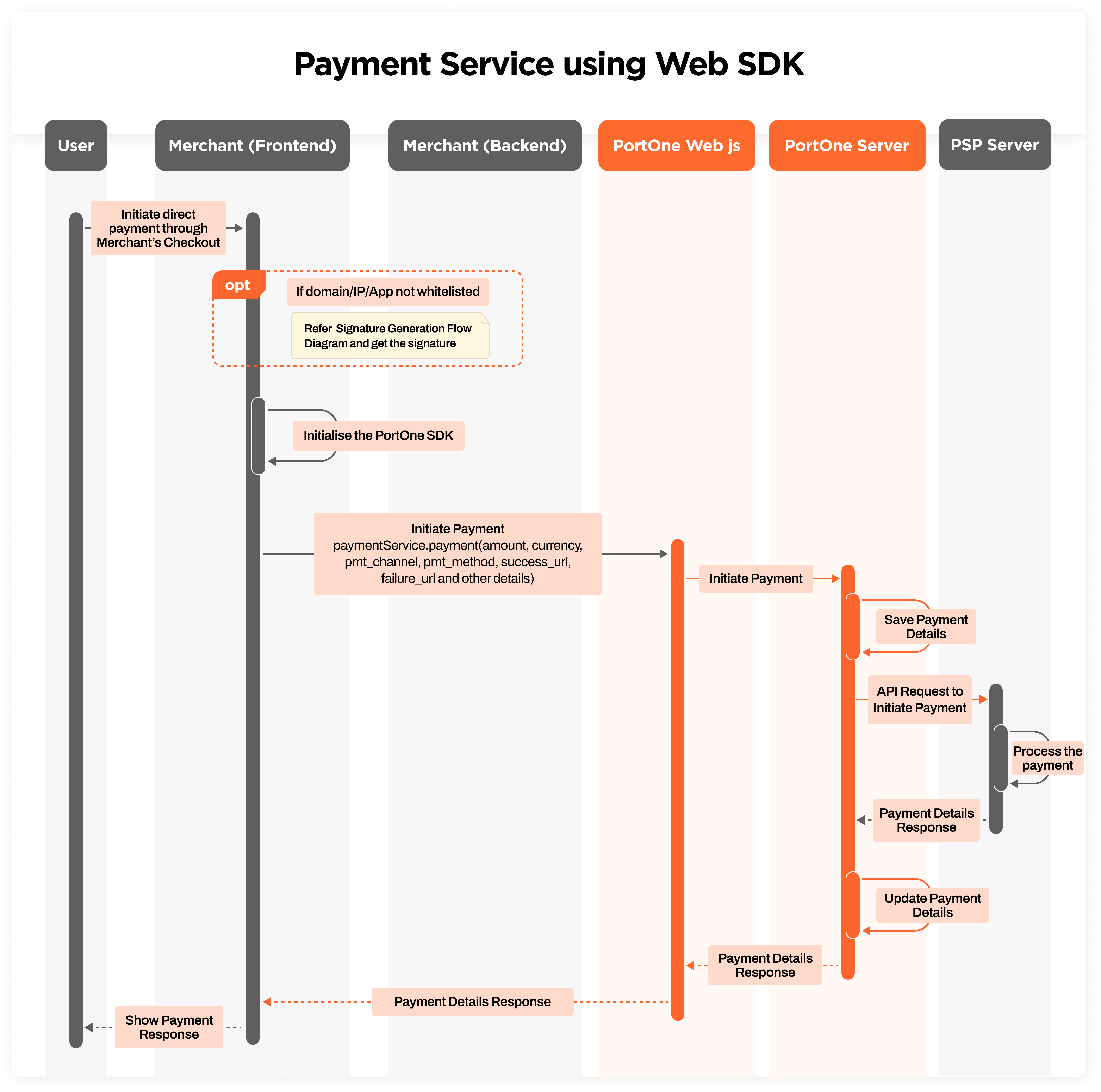
Once the user initiates a payment via the Merchant's Checkout:
- The merchant initializes the PortOne SDK and calls PortOne Web JS with the required payment details.
- PortOne Server saves the payment details, sends the request to the PSP Server, and processes the payment.
- The PSP Server returns the payment details, which PortOne pre-processes and converts into a standard response object.
- The response flows back to the merchant’s front end, which can display the result to the user.
Passing the signature hash can be skipped if you whitelist your domain/IP/App
Recipes for a quick start
To generate the signature hash, you can also refer to Generate Signature Hash.
Sample code
Use the following JS code snippet to initiate the payment using the respective supported payment channels (e.g. MOMO, ZaloPay, Mastercard, etc).
<script>
const portone = new window.PortOne({
// Your PortOne Key
portOneKey: 'pptafmcddmszvgXl'
})
portone.paymentService.payment({
// new uniform payment request format example for Momopay
"key": "pptafmcddmszvgXl",
"pmt_channel": "STRIPE",
"pmt_method": "STRIPE_CARD",
"merchant_order_id":"MERCHANT1617366877238",
"signature_hash": "10b664f803035a2146b26949041c2ce8c8693512e4b85159d3c43b001a714af0",
"amount": 4000,
"currency": "VND",
"environment": "live",
"description": "Product description",
"response_type": "redirect_url_only",
"success_url": "https://checkout.portone.cloud/success.html",
"failure_url": "https://checkout.portone.cloud/failure.html"
})
</script>
Detailed script for payment service (including optional fields.)
<script>
const portone = new window.PortOne({
// Your PortOne Key
portOneKey: 'pptafmcddmszvgXl'
})
portone.paymentService.payment({
// new uniform payment request format example for Momopay
"key": "pptafmcddmszvgXl",
"pmt_channel": "STRIPE",
"pmt_method": "STRIPE_CARD",
"merchant_order_id":"MERCHANT1617366877238",
"signature_hash": "10b664f803035a2146b26949041c2ce8c8693512e4b85159d3c43b001a714af0",
"amount": 4000,
"currency": "VND",
"environment": "live",
"description": "Product description",
"billing_details": {
"billing_name": "Mark Weins",
"billing_email": "[email protected]",
"billing_phone": "1234567890",
"billing_address": {
"city": "Ho Chi Minh City",
"country_code": "VN",
"locale": "en",
"line_1": "address_1",
"line_2": "address_2",
"postal_code": "400202",
"state": "Mah"
}
},
"shipping_details": {
"shipping_name": "Mark Weins",
"shipping_email": "[email protected]",
"shipping_phone": "1234567890",
"shipping_address": {
"city": "Ho Chi Minh City",
"country_code": "VN",
"locale": "en",
"line_1": "address_1",
"line_2": "address_2",
"postal_code": "400202",
"state": "Mah"
}
},
"order_details": [
{
"id": "1",
"price": 2000,
"name": "Stubborn Attachments",
"quantity": 1,
"image":"https://cdn.portone.cloud/item1.png"
},
{
"id": "2",
"price": 2000,
"name": "Stubborn Attachments",
"quantity": 1,
"image":"https://cdn.portone.cloud/item2.png"
}
],
"response_type": "redirect_url_only",
"success_url": "https://checkout.portone.cloud/success.html",
"failure_url": "https://checkout.portone.cloud/failure.html",
"pending_url": "https://checkout.portone.cloud/pending.html"
})
</script>
Parameter list for Payment Service
key
key
string · required
The unique PortOne key for merchant
merchant_order_id
merchant_order_id
string · required
The unique merchant order reference generated by the merchant
pmt_channel
pmt_channel
string · required
The Payment Channel Key is listed in PortOne docs
pmt_method
pmt_method
string · required
The Payment Method Key listed in PortOne docs
description
description
string · required
The description of the transaction
environment
environment
string · required
The environment of the transaction is either live OR sandbox
amount
amount
double · required
The amount of the transaction can be a floating-point number
currency
currency
string · required
The currency of the transaction
signature_hash
signature_hash
string · required
The signature hash of transaction details generated as per docs
billing_details
billing_details
object
The JSON object for billing details
billing_name
string · The billing first and middle name
billing_surname
string · The billing last name
billing_email
string · The billing email address
billing_phone
string · The billing phone number
billing_address
###### objectThe JSON object containing full address
city
string · City name
country_code
string · 2 digit country code
country_name
string · Full country name
locale
string · Region locale
line_1
string · Line 1 of the address
line_2
string · Line 2 of the address
postal_code
string · Postal code of the area
state
string · State/province of the country
shipping_details
shipping_details
object
The JSON object for shipping details
shipping_name
string · The shipping first and middle name
shipping_surname
string · The shipping last name
shipping_email
string · The shipping email address
shipping_phone
string · The shipping phone number
shipping_address
object ·The JSON object containing full address
city
string · City name
country_code
string · 2 digit country code
country_name
string · Full country name
locale
string · Region locale
line_1
string · Line 1 of the address
line_2
string · Line 2 of the address
postal_code
string · Postal code of the area
state
string · State/province of the country
token_params
token_params
object
The JSON object for initiating transactions using card details
token
string · The token details by PortOne
partial_card_number
string · Partial card number used for identification purposes
expiry_month
string · The expiration month of the card
expiry_year
string · The expiration year of the card
type
string · Type of card (e.g. Visa, Mastercard)
save_card
boolean · Whether to save the card for future use
is_channel_token
boolean · Whether the token is a channel token, the default is false. Used for non-seamless card transactions.
order_details
order_details
array of objects
The JSON array for order details
id
string · The unique identifier of the order-item
name
string · The name of the product
price
double · The unit price of the product
quantity
integer · The quantity of the product
image
string · The URL of the product image
additional_details
object · Additional details about the order (This varies as per PSP specification)
success_url
success_url
string · required
The URL of the success page hosted by the merchant
failure_url
failure_url
string · required
The URL of the failure page hosted by the merchant
pending_url
pending_url
string
The URL of the pending page hosted by the merchant
routing_enabled
routing_enabled
boolean
Boolean flag to identify if routing is enabled
routing_params
routing_params
object
The JSON object for adding routing details, is only applicable if routing is enabled
type
string · The type of routing ·
routing_ref
string · The route ID that is created from the Admin Dashboard
data
array of objects
transaction_type
transaction_type
string
The type of the transaction to capture (e.g., PURCHASE)
override_auto_redirect
override_auto_redirect
boolean
Boolean flag to abort auto redirection and use the response
user_configured_field1
user_configured_field1
string
Custom user-configured field 1
user_configured_field2
user_configured_field2
string
Custom user-configured field 2
user_configured_field3
user_configured_field3
string
Custom user-configured field 3
user_configured_field4
user_configured_field4
string
Custom user-configured field 4
user_configured_field5
user_configured_field5
string
Custom user-configured field 5
Updated 2 months ago