Checkout Service
The Checkout Service provides a pre-built, hosted checkout page that simplifies the payment process for businesses. The Checkout Service is much easier to integrate, requiring minimal code and effort from the merchant’s side. It’s designed for businesses that want to avoid the complexity of building and managing a custom payment flow.
Hosted Payment Flow
With the Checkout Service, users are redirected to a secure, hosted checkout page maintained by PortOne, where they can complete the transaction. After payment, they are redirected back to the merchant's site or app.
Ideal for Quick Setup
This service is best suited for businesses or applications that need a payment solution up and running quickly without the need for deep customization or development resources.
Customization Options
Unlike the Payment Service, which allows for fully customized, branded checkouts, the Checkout Service is designed to be fast and easy to integrate.
While the Checkout Service is more limited in terms of customization compared to the Payment Service, merchants can still adjust certain elements such as branding (logo, colors) to ensure a consistent look.
Use Case
Ideal for small to medium businesses or apps that prioritize speed of integration over full control of the user experience.
Integration flow
The sequence diagram below illustrates the flow for integrating the Checkout service via the Web SDK
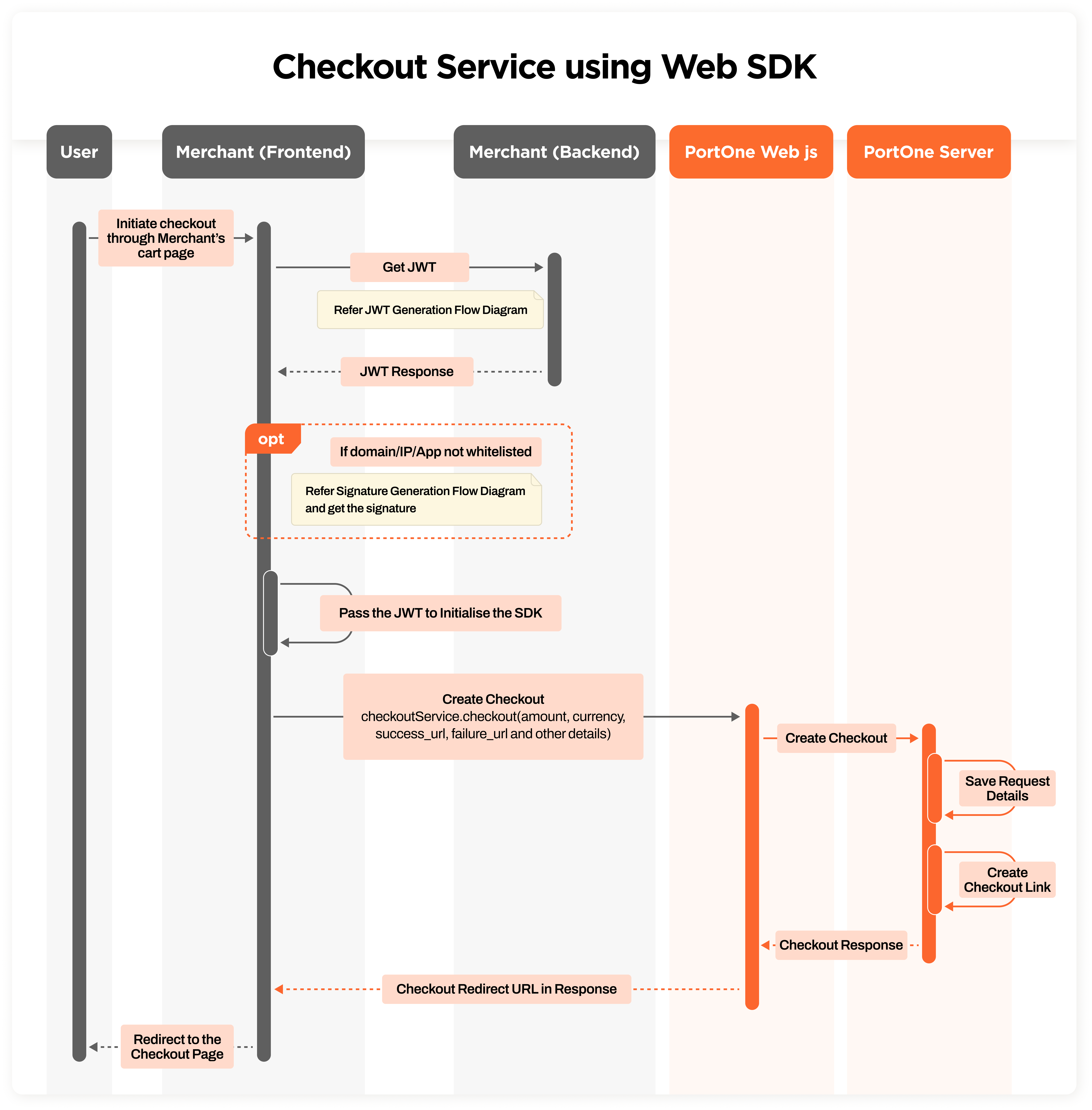
Once the user initiates checkout via the Merchant's cart page:
- The Merchant (front end) requests a JWT from the Merchant (Back end)
- The Merchant (front end) passes the JWT to initialize the PortOne SDK and then calls PortOne Web JS to create the checkout with payment details.
- PortOne Server saves the request details, generates the checkout link, and returns it to the Merchant (front end).
- Merchants can use this checkout link to redirect the user
Passing the signature hash can be skipped if you whitelist your domain/IP/App
Recipes for a quick start
To generate the signature hash, you can also refer to Generate Signature Hash.
To generate the JWT token, you can also refer to Generate JWT Token.
Sample code
Use the following JS code snippet to initiate the payment in the checkout service using the respective supported payment channels (e.g. MomoPay, ZaloPay, Mastercard, etc).
<script>
const portone = new window.PortOne({
// Your PortOne Key
portOneKey: 'pptafmcddmszvgXl',
jwtToken: 'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiJOUFNrWlpZZWZHeUt2QnhpIiwiaXNzIjoiQ0hBSVBBWSIsImlhdCI6MTYzMzMzOTAzMiwiZXhwIjoxNjczMzM5MDMy',
})
portone.checkoutService.checkout({
"merchant_details": {
"name": "Downy",
"logo": "images/v184_135.png",
"back_url": "https://demo.chaiport.io/checkout",
"promo_code": "Downy350",
"promo_discount": 0.0,
"shipping_charges": 0.0,
},
"merchant_order_id":"MERCHANT1617366877238",
"signature_hash": "10b664f803035a2146b26949041c2ce8c8693512e4b85159d3c43b001a714af0",
"amount": 4000,
"currency": "VND",
"environment": "live",
"description": "Product description",
"country_code": "VN",
"expiry_hours":24,
"is_checkout_embed": true,
"show_back_button": false,
"show_shipping_details": false,
"default_guest_checkout": true,
"show_items": false,
"success_url": "https://checkout.portone.cloud/success.html",
"failure_url": "https://checkout.portone.cloud/failure.html"
})
</script>
Detailed script for checkout service (including optional fields.)
<script>
const portone = new window.PortOne({
// Your PortOne Key
portOneKey: 'pptafmcddmszvgXl',
jwtToken: 'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiJOUFNrWlpZZWZHeUt2QnhpIiwiaXNzIjoiQ0hBSVBBWSIsImlhdCI6MTYzMzMzOTAzMiwiZXhwIjoxNjczMzM5MDMy',
})
portone.checkoutService.checkout({
"merchant_details": {
"name": "Downy",
"logo": "images/v184_135.png",
"back_url": "https://demo.chaiport.io/checkout",
"promo_code": "Downy350",
"promo_discount": 0.0,
"shipping_charges": 0.0,
},
"merchant_order_id":"MERCHANT1617366877238",
"signature_hash": "10b664f803035a2146b26949041c2ce8c8693512e4b85159d3c43b001a714af0",
"amount": 4000,
"currency": "VND",
"environment": "live",
"description": "Product description",
"order_details": [{
"id":"1",
"price": 2000,
"name": "Stubborn Attachments",
"quantity": 1
},
{
"id":"2",
"price": 2000,
"name": "Stubborn Attachments",
"quantity": 1
}
],
"billing_details": {
"billing_name": "Test mark",
"billing_email": "[email protected]",
"billing_phone": "9998878788",
"billing_address": {
"city": "VND",
"country_code": "VN",
"locale": "en",
"line_1": "address",
"line_2": "address_2",
"postal_code": "400202",
"state": "Mah"
}
},
"shipping_details": {
"shipping_name": "xyz",
"shipping_email": "[email protected]",
"shipping_phone": "1234567890",
"shipping_address": {
"city": "abc",
"country_code": "VN",
"locale": "en",
"line_1": "address_1",
"line_2": "address_2",
"postal_code": "400202",
"state": "Mah"
}
},
"country_code": "VN",
"expiry_hours":24,
"is_checkout_embed": true,
"show_back_button": false,
"show_shipping_details": false,
"default_guest_checkout": true,
"show_items": false,
"success_url": "https://checkout.portone.cloud/success.html",
"failure_url": "https://checkout.portone.cloud/failure.html",
"use_merchant_configured_dcc_rate": true,
"merchant_dcc_rate": {
"forex_rate": 0.000038,
"invoice_amount": 4000,
"invoice_currency": "VND",
"payment_currency": "USD",
"payment_amount": 0.15,
"show_fx_conversion": true
}
})
</script>
Parameter list for Checkout Service
merchant_details
merchant_details
object · required
The JSON object for merchant details
name
· required
string · The name of the merchant
logo
string · The logo of the merchant
back_url
string · The url of the merchant site
promo_code
string · The promo code enabled on the order by the merchant
promo_discount
· required
double · The promo code discount amount on the order by the merchant
shipping_charges
· required
number · The shipping charges set by the merchant
merchant_order_id
merchant_order_id
string · required
The unique merchant order reference generated by the merchant
signature_hash
signature_hash
string · required
The signature hash of transaction details generated as per docs
environment
environment
string · required
The environment of the transaction is either live OR sandbox
amount
amount
double · required
The amount of the transaction can be a floating-point number
currency
currency
string · required
The currency of the transaction
country_code
country_code
string · required
The country code of the transaction
description
description
string · required
The description of the transaction
billing_details
billing_details
object
The JSON object for billing details
billing_name
string · The billing first and middle name
billing_surname
string · The billing last name
billing_email
string · The billing email address
billing_phone
string · The billing phone number
billing_address
objectThe JSON object containing full address
city
string · City name
country_code
string · 2 digit country code
country_name
string · Full country name
locale
string · Region locale
line_1
string · Line 1 of the address
line_2
string · Line 2 of the address
postal_code
string · Postal code of the area
state
string · State/province of the country
shipping_details
shipping_details
object
The JSON object for shipping details
shipping_name
string · The shipping first and middle name
shipping_surname
string · The shipping last name
shipping_email
string · The shipping email address
shipping_phone
string · The shipping phone number
shipping_address
object ·The JSON object containing full address
city
string · City name
country_code
string · 2 digit country code
country_name
string · Full country name
locale
string · Region locale
line_1
string · Line 1 of the address
line_2
string · Line 2 of the address
postal_code
string · Postal code of the area
state
string · State/province of the country
order_details
order_details
array of objects
The JSON array for order details
id
string · The unique identifier of the order-item
name
string · The name of the product
price
double · The unit price of the product
quantity
integer · The quantity of the product
image
string · The URL of the product image
additional_details
###### object · Additional details about the order (This varies as per PSP specification)
success_url
success_url
string · required
The URL of the success page hosted by the merchant
failure_url
failure_url
string · required
The URL of the failure page hosted by the merchant
pending_url
pending_url
string
The URL of the pending page hosted by the merchant.
expiry_date
expiry_date
string
Date and time to expire the payment link in UTC format.
source
source
string · required
'default', 'api', or 'checkout' -- The Source of Payment Link creation.
show_shipping_details
show_shipping_details
boolean
The boolean flag will show the shipping details on the Checkout page.
show_back_button
show_back_button
boolean
A boolean flag will show the back button on the Checkout page.
default_guest_checkout
default_guest_checkout
boolean
Boolean flag to enable default guest checkout
is_checkout_embed
is_checkout_embed
boolean
Boolean flag to enable checkout embedding
notify_by_email
notify_by_email
boolean
Boolean flag to notify the customer by email
notify_by_phone
notify_by_phone
boolean
Boolean flag to notify customers by phone
override_auto_redirect
override_auto_redirect
boolean
Boolean flag to abort auto redirection and use the response
user_configured_field1
user_configured_field1
string
Custom user-configured field 1
user_configured_field2
user_configured_field2
string
Custom user-configured field 2
user_configured_field3
user_configured_field3
string
Custom user-configured field 3
user_configured_field4
user_configured_field4
string
Custom user-configured field 4
user_configured_field5
user_configured_field5
string
Custom user-configured field 5
use_merchant_configured_dcc_rate
use_merchant_configured_dcc_rate
boolean
Boolean flag to configure the payment link to use the merchant's configured DCC rates in case of DCC checkout
merchant_dcc_rate
merchant_dcc_rate
forex_rate
· required
float · The currency conversion forex rate
invoice_amount
required
float · The actual invoice amount of your purchase
invoice_currency
required
string · The actual invoice currency of your purchase
payment_amount
required
float · The payment amount of your purchase after applying the forex conversation rate
payment_currency
required
string · The payment currency of your purchase
show_fx_conversion
boolean · The boolean flag to show fx rate in the checkout page
Embedding the checkout using iFrame
Embedding a checkout experience within an iFrame provides seamless, uninterrupted payment flows by allowing the checkout interface to load directly on your page. Instead of redirecting customers, the embedded checkout offers an improved user experience by keeping users on your website. Below are the key steps and necessary configuration details to implement the embedded checkout correctly.
Step 1: Configure Checkout Payload Settings
To ensure the checkout service behaves appropriately for embedding, you must set the following flags in your checkout payload:
is_checkout_embed: true
override_auto_redirect: false
Step 2: Adding the iFrame to Your Page
The following HTML code snippet must be added to your webpage to load the embedded checkout UI. This structure ensures that the checkout interface displays properly with appropriate styling, z-index management, and backdrop handling.
<div
class="portone-container"
id="portone-container"
style="z-index: 1000000000;position: fixed;top: 0px;display: none;left: 0px;height: 100%;width: 100%;backface-visibility: hidden;overflow-y: visible;"
>
<div
class="portone-backdrop"
style="min-height: 100%; transition: all 0.3s ease-out 0s; position: fixed; top: 0px; left: 0px; width: 100%; height: 100%; background: rgba(0, 0, 0, 0.5);"
></div>
<iframe
style="opacity: 1; height: 100%; position: relative; background: none; display: block; border: 0px none transparent; margin: 0px; padding: 0px; z-index: 2; width: 100%;"
allowtransparency="true"
frameborder="0"
width="100%"
height="100%"
allowpaymentrequest="true"
src=""
id="portone-checkout-frame"
class="portone-checkout-frame"
></iframe>
</div>;
Step 3: Using the Checkout Service
Once the above code is added to the page, you can implement the checkout service.
Key Considerations
- Cross-Origin Restrictions
Ensure your iFrame source (src URL) is from a trusted domain to avoid CORS (Cross-Origin Resource Sharing) issues. - Browser Compatibility
While most modern browsers support iFrame embedding, certain restrictions on cookies or third-party scripts may affect the checkout experience. Ensure thatallowpaymentrequest
is enabled for secure payments.
Updated 12 days ago